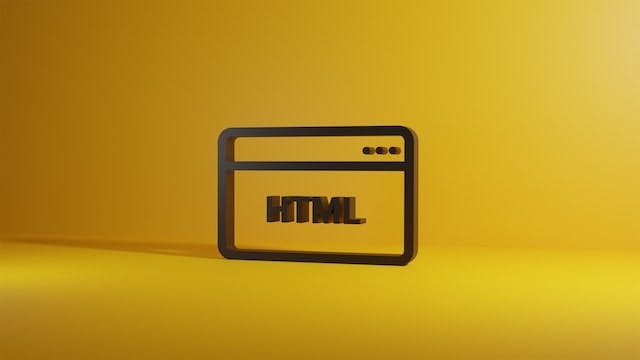
Optimizing Next.js App Performance for Speed and SEO
Introduction
Welcome to our article on optimizing your Next.js app's performance through the use of getServerSideProps and getStaticProps. As a big NextJS fan, I want to show how can we get the best results using NextJS's power.
In this article, we will delve into the details of these two methods and show you how to use them effectively to fetch data in the most efficient way possible. Whether you're a seasoned Next.js developer or just getting started, this article will provide you with the knowledge you need to take your app's performance to the next level. So, let's get started and see how we can use these powerful tools to improve the speed and functionality of your Next.js app.
The importance of optimizing the Next.js app's performance
When it comes to building web applications, performance is key. Nobody wants to use a slow and clunky app, and in today's fast-paced world, people expect websites to load quickly and smoothly. This is especially true when it comes to Next.js, a popular framework for building React apps. With Next.js, you can build powerful and efficient web apps, but it's important to take the time to optimize your app's performance to ensure that it runs at its best.
What is the meaning of Optimization
Optimizing your Next.js app's performance can mean the difference between a successful app and one that falls short. It can also mean the difference between a good user experience and a frustrating one. And let's not forget about SEO, search engines like Google will also reward the fast and optimized websites with better ranking which will bring more traffic to your website. If you're working with Next.js, you might have heard of this fancy feature called incremental static regeneration. Basically, it's a way for Next.js to optimize the performance of your web app by only regenerating the parts that have changed, instead of the whole thing every time. Sounds pretty cool, right?
Let's break down getServerSideProps and getStaticProps, and how they can help you optimize your Next.js app's performance.
Understanding getServerSideProps
getServerSideProps is a method that lets you fetch data on the server-side during the initial request. This is perfect for situations where the data being fetched is specific to the user, or when the data is dynamic and changes often.
Here's a simple example of how you might use getServerSideProps to fetch a user's profile information:
export async function getServerSideProps(context) {
// Fetch user's profile information
const res = await fetch(`https://my-api.com/users/${context.params.userId}`);
const user = await res.json();
// Pass user data to the page component
return { props: { user } }
}
Understanding getStaticProps
On the other hand, getStaticProps is a method that lets you fetch data at build time, and the data is then stored as a JSON file. This is perfect for situations where the data being fetched is static, and doesn't change often.
Here's an example of how you might use getStaticProps to fetch a list of blog posts:
export async function getStaticProps() {
const slug = context.params?.slug as string;
const blogPosts = await client.getEntries({
'fields.slug[in]': slug,
});
const blogPost = blogPosts.items[0].fields as IBlogPostFields;
return {
props: {
blogPost,
},
};
}
To be able to use getStaticProbs, we need to specify all the paths of pages that we want to create statically. So, with getStaticPaths function we are defining all the paths.
export async function getStaticPaths(){
const allPosts = await client.getEntries();
const paths = allPosts.items.map((post) => {
return {
params: { slug: post.slug },
};
});
return {
paths,
fallback: 'blocking',
};
}
The differences between getServerSideProps and getStaticProps
So, the main difference between getServerSideProps and getStaticProps is that the former is executed on the server-side during the initial request and the latter is executed during the build time.
It's important to keep in mind that, getStaticProps is only called at build time, whereas getServerSideProps is called on each request, so it's better to use it for dynamic data, and use getStaticProps for static data, this way you can get the best performance out of your Next.js app.
Improving the getStaticProps with On Deman Revalidation
One way you can take advantage of this is by using on-demand revalidation. It's a fancy way of saying that you can selectively revalidate certain parts of your app, without having to regenerate the whole thing. You can do this using the getStaticProps and getServerSideProps methods, which let you specify which data needs to be revalidated.
So, we said that static pages are only generated when the build time. But what if we want to serve these pages dynamically and frequently? NextJS
There is a great feature for revalidating static page content using the NextJS API directory. Here is the official example from NextJS:
// pages/api/revalidate.js
export default async function handler(req, res) {
// Check for secret to confirm this is a valid request
if (req.query.secret !== process.env.MY_SECRET_TOKEN) {
return res.status(401).json({ message: 'Invalid token' })
}
try {
// this should be the actual path not a rewritten path
// e.g. for "/blog/[slug]" this should be "/blog/post-1"
await res.revalidate('/path-to-revalidate')
return res.json({ revalidated: true })
} catch (err) {
// If there was an error, Next.js will continue
// to show the last successfully generated page
return res.status(500).send('Error revalidating')
}
}
I found the best way of revalidating the posts for Contentful Headless CMS is creating a webhook while creating, publishing, and updating. With this way, we can trigger the revalidating functionality in the background.
In Conclusion
Optimizing the performance of your Next.js app is crucial for building a successful and efficient web application. By using features like getServerSideProps and getStaticProps, you can improve the speed and performance of your app, and make sure that it's running at its best. Remember that performance is not only important for user experience but also for SEO, faster website means better ranking and more traffic. By following the best practices outlined in this article, you can take your Next.js app to the next level and ensure that it's running at its peak performance.
Note: This article prepared with help of ChatGPT