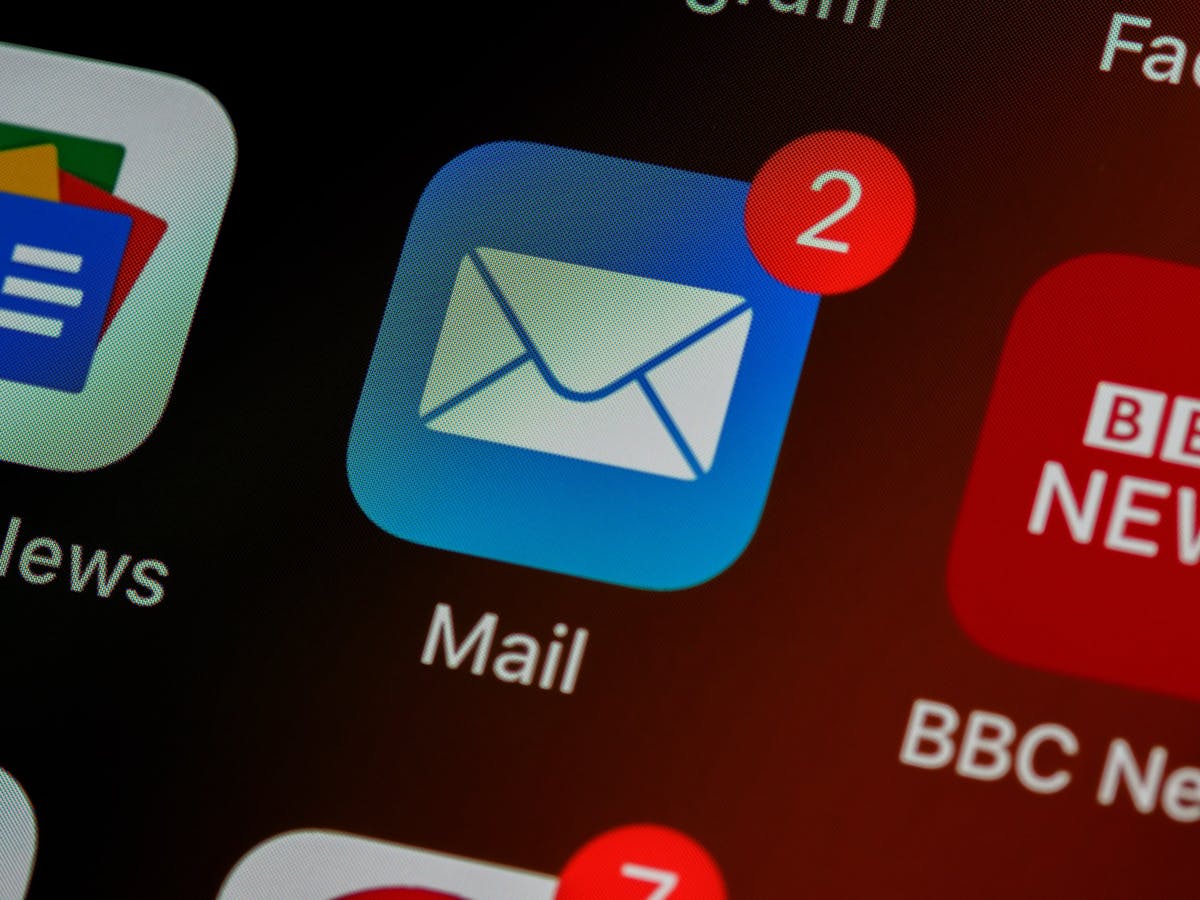
Sending Emails with Ease: A Step-by-Step Guide to Using Nodemailer with TypeScript in Next.js
About Nodemailer
Nodemailer is a popular library for sending emails in Node.js. It allows you to send emails using various transport methods, such as SMTP, AWS SES, and more. In this post, we will be using Nodemailer with TypeScript to send emails from a Next.js application.
Before diving into the code example, let's go over some key concepts of Nodemailer. Nodemailer is a module for Node.js applications to send emails. It supports various transport methods such as SMTP, AWS SES, and more. With Nodemailer, you can send plaintext or HTML emails, attachments, and more.
Use Cases for Nodemailer
Nodemailer is a versatile library that can be used in a variety of different use cases. Some common use cases include:
-
Sending confirmation emails: When a user signs up for a service or makes a purchase, a confirmation email can be sent to their email address to confirm the action.
-
Sending password reset emails: When a user forgets their password, a password reset link can be sent to their email address to allow them to reset their password.
-
Sending notifications: Applications can send notifications to users via email, such as updates on their account or new features that have been added.
-
Sending marketing emails: Applications can send marketing emails to users, such as newsletters or promotional offers.
-
Sending bulk emails: Applications can send bulk emails to a large number of users at once, such as in the case of a mass mailing campaign.
-
Sending automated emails: Applications can send automated emails in response to certain events or actions, such as when a user's account is about to expire.
-
Send HTML email Templates: Applications can use Nodemailer to send HTML email Templates, which can be designed and customized to match the look and feel of the application.
-
Sending emails with attachments: Applications can use Nodemailer to send emails with attachments, such as invoices, receipts, or other files.
These are just a few examples of the many use cases for Nodemailer, it is a very flexible library that can be used in many different ways to meet the needs of your application.
In this post, we'll be using the SMTP transport method to send emails using a Gmail account. We will be using TypeScript to write our code, which adds type safety and improved code organization to our application.
Now that we understand the basics of Nodemailer and TypeScript, let's take a look at the code example.
Implementation of Emailer Class
The code below shows an implementation of the Nodemailer library in TypeScript. The Emailer class is used to send emails using a Gmail account. It uses the nodemailer.createTransport()
method to create a transporter object, which is used to send emails.
import * as nodemailer from "nodemailer";
import { MailOptions } from "nodemailer/lib/json-transport";
export class Emailer {
private readonly transporter: nodemailer.Transporter;
constructor() {
this.transporter = nodemailer.createTransport({
service: "gmail",
auth: {
user: process.env.GMAIL_USER,
pass: process.env.GMAIL_PASSWORD,
},
});
}
public sendEmail(mailOptions: MailOptions) {
return this.transporter.sendMail(mailOptions);
}
public notifyAdminForNewUser(email: string, username: string) {
this.sendEmail(notifyAdminNewUserEmailTemplate(email, username));
}
public notifyUserForSignup(email: string, username: string) {
this.sendEmail(newUserEmailTemplate(email, username));
}
}
export const emailer = new Emailer();
export const newUserEmailTemplate = (email: string, username: string) => {
return {
from: process.env.GMAIL_USER,
to: email,
subject: `${username}, Welcome to the our website`,
text: "Welcome to the our website",
html: `
<h1>Welcome to our website!</h1>
<p>We're glad you've decided to join us. We hope you find everything you're looking for here and enjoy using our site.</p>
<p>If you have any questions or need any help, please don't hesitate to contact us. Thank you for signing up!</p>
`,
} as MailOptions;
};
export const notifyAdminNewUserEmailTemplate = (
email: string,
username: string
) => {
return {
from: process.env.GMAIL_USER,
to: process.env.GMAIL_USER,
subject: `New User: ${username} - email: ${email}`,
text: `New User: ${username} - email: ${email}`,
html: `
<h1>New User: ${username}</h1>
<p>email: ${email}</p>
`,
} as MailOptions;
};
The class has two methods, notifyAdminForNewUser
and notifyUserForSignup
, which use the sendEmail
method to send emails to the new user and the admin, respectively. The sendEmail
method takes in a mailOptions object, which contains the recipient's email, subject, and the message.
The newUserEmailTemplate
and notifyAdminNewUserEmailTemplate
functions are used to create the mailOptions
object for the new user and admin emails, respectively. They take in the user's email and username as arguments and return an object with the recipient's email, subject, and message.
It's worth noting that the code is using process.env.GMAIL_USER
and process.env.GMAIL_PASSWORD
to authenticate with gmail
, You should replace it with your own Gmail user and password.
To obtain the Gmail password, you should go to the settings on Gmail and create an App Password, please remember that you need to activate the 2FA for your google account.
Conclusion
Nodemailer with TypeScript makes it easy to send emails from a Next.js application. With the help of the Emailer
class and the mailOptions
object, you can send emails with ease. Of course, this implementation will only work on the server side of the NextJS Application. If you want to send email from client side, you just need to create an API endpoint under the /pages/api
directory.
In this post, we've shown how to send emails using a Gmail account, but you can also use other transport methods such as AWS SES to send emails. You can expand the class methods to meet your needs.