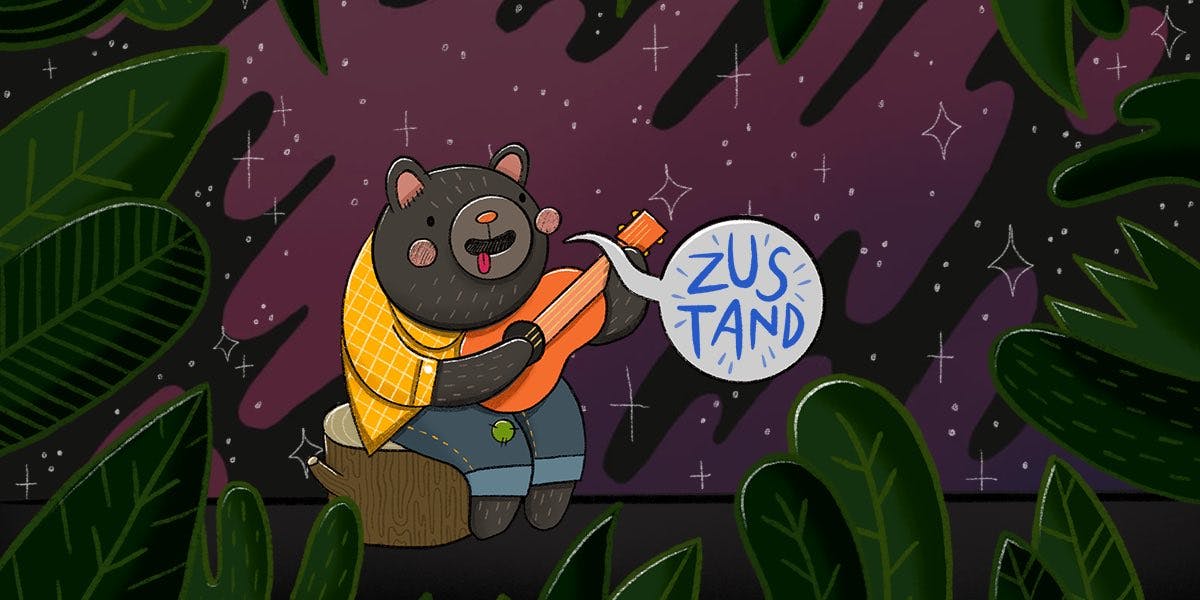
Zustand - Create a Store for State Management with Typescript
#nextjs
#react
#state
#zustand
Simple usage of Zustand State Management
Import the creation from zustand:
import { create } from "zustand"
Define the states with an interface:
interface Props {
count: number;
increaseCount: () => void;
decreaseCount: () => void;
}
Create a custom hook with the 'create' method. There are many middlewares that we can use like persist for localStorage, devtools for enabling redux developer tool, etc.
export const useStore = create<Props>()(
(set, get) => (
{
count: 0,
increaseCount() {
set({ count: get().count + 1 });
},
decreaseCount() {
const state = get();
set({ count: state.count - 1 });
}
}
)
)
Using of the hook in a Component:
const count = useStore(state => state.count);
const increaseCount = useStore(state => state.increaseCount);
const decreaseCount = useStore(state => state.decreaseCount);
Note: No Provider needed 👍